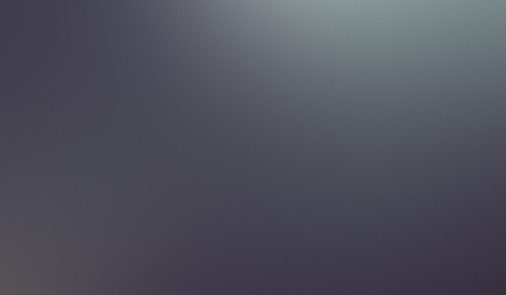
Dataflow Analysis
Explorer the Dataflow Analyzer which forms the core capability of wSAST.
Learn moreFlexible static code analysis framework for consultants and developers.
The wSAST framework supports purely static analysis in addition to dataflow analysis. In this mode the analyzer performs checks only against the AST structure of the code being analysed rather than following execution flows. This means that findings can lack a clear path to exploitation, but scans are completed extremely quickly in comparison, and provide a good starting point for manual review while dataflow analysis is being performed.
Rules are either written against the provided Common Rules Engine using an XML-based format which enables functions, variables, types and data source and sinks to be described easily without code, or can be imported as custom .NET plugins implementing the appropriate interface.
A near identical format as used for Common Rules Engine sources and sinks is employed, enabling checks written as dataflow analyzer checks to be reused as static analyzer checks when using the XML format. An example of the Common Rules Engine static analysis rule format includes:
<function name="MemCpySink" languages="wsil, c" report="true" categories="MEM_CORRUPTION" title="Potentially dangerous copy operation." description="The function has been known to lead to memory corruption vulnerabilities when incorrectly employed; review usage.">
<signature names="MemCpy, memcpy, CopyMemory, RtlCopyMemory, memmove" param-count="3" />
<param pos="1" name="dst" types="uint8\[\]"/>
<param pos="2" name="src" types="uint8\[\]"
<param pos="3" name="len" types="int32"
</function>
This can be used to define functions as sinks, specifying parameter types, counts, alternative names, arbitrary categories of vulnerability they can cause (e.g. "MEM_CORRUPTION"), as well as whether the function is a member of any class or particular namespace and whether it is virtual or not (if it is then the class hierarchy will be searched for each potential instance). This differs from the Common Rules XML format for dataflow analysis only in that variable links cannot be checked, and the traced property is redundant.
<variable name="WriteToPasswordVariable" languages="*" report="true" categories="MEM_CORRUPTION" title="Writes to a variable named password." description="The code writes to a local or member variable named password." >
<definition prefix-types="UserModel, .*" prefix-virtual="true" types=".*" virtual="true" names="[pP]ass(wd|word)?" access="write" traced="true" />
</variable>
This can be used to define variables or data members which can act as sinks, for example specific fields of a Crypto object for example specifying the cipher in use, or fields such as those named "Password". The variable or member name is matched using regular expressions, as is the containg class or namespace. The access type (read or write) can also be configured, and for the "write" case whether the variable on the right hand side of the assignment should be traced for the sink to trigger. For type comparison class hierarchies can also be considered if virtual.
<data name="SinkInsertOrUpdate" languages="*" report="true" categories="SQL_INJECTION" title="SQL Query Sink" description="Writes data containing a SQL query">
<definition types="string" value="(SELECT|UPDATE)\s+.*" traced="true" />
</data>
This can be used to define data values which act as a sink when combined with a traced input, for example a fragment of an SQL statement being concatenated to a user-supplied input. Regular expressions are used to match values.
For complete control over the implementation of a particular rule, for example to implement a rule which is not based on matching function, variable or data usage, it is possible to implement custom rules entirely in .NET. The following interface is provided for this purpose:
public interface IStaticRule
{
string GetRuleName();
string GetRuleApplicableNodeType();
string GetRuleApplicableNodeName();
IStaticRuleResult PerformCheck(WrappedWsilNode node);
}
The assembly containing the implementation is imported via the wSAST configuration file, and then for each node encountered during dataflow analysis if the node type matches the output of GetRuleApplicableNodeType()
(or if this returns null, all nodes types), and if specified the node name matches the output of GetRuleApplicableNodeName()
then the PerformCheck()
method is invoked.
If the returns PerformCheck()
returns a valid IStaticRuleResult
instance then a finding is reported. The PerformCheck()
has full access to the affected WSIL node and all parent/child nodes to implement a rule.
The static analyzer can be configured to execute with resource constraints, for example the number of threads specified for simultaneous analysis. By design the static analyzer uses very minimal memory while running.
Reporting is performed through an interface which enables reports to be generated for any format desired; the plugins are configured within the wSAST XML configuration file. The provided reporting plugin writes code to a text file with a name which can be customised based on source/sink name, finding category, date, and other factor. Either entire traces (as below) or partial traces can be logged.
...
Title: Potential Arbitrary File Read
Rule: load
Category: ALL_CATEGORIES
Description: Arbitrary files can be read by manipulating the loaded properties.
Source File: C:\wsast\examples\JavaVulnerableLab-master\src\main\java\org\cysecurity\cspf\jvl\controller\Install.java (line 65)
config.load(new FileInputStream(configPath));
----------------------------------------------------------------
Title: Potential Open Redirect Vulnerability
Rule: sendRedirect
Category: ALL_CATEGORIES
Description: Using sendRedirect to perform a redirect to a user-controlled URL
Source File: C:\wsast\examples\JavaVulnerableLab-master\src\main\java\org\cysecurity\cspf\jvl\controller\Logout.java (line 40)
response.sendRedirect("index.jsp");
}
----------------------------------------------------------------
Title: Potential Security Vulnerability
Rule: addCookie
Category: ALL_CATEGORIES
Description: Using addCookie to add a cookie to the HTTP response
Source File: C:\wsast\examples\JavaVulnerableLab-master\src\main\java\org\cysecurity\cspf\jvl\controller\LoginValidator.java (line 60)
response.addCookie(privilege);
----------------------------------------------------------------
Title: Potential SQL Injection
Rule: executeQuery
Category: ALL_CATEGORIES
Description: Using executeQuery without parameter binding
Source File: C:\wsast\examples\JavaVulnerableLab-master\src\main\java\org\cysecurity\cspf\jvl\controller\EmailCheck.java (line 48)
rs=stmt.executeQuery("select * from users where email='"+email+"'");
----------------------------------------------------------------
Title: Potential Open Redirect Vulnerability
Rule: sendRedirect
Category: ALL_CATEGORIES
Description: Using sendRedirect to perform a redirect to a user-controlled URL
Source File: C:\wsast\examples\JavaVulnerableLab-master\src\main\java\org\cysecurity\cspf\jvl\controller\Open.java (line 39)
response.sendRedirect(url);
}
else
----------------------------------------------------------------
Title: Potential SQL Injection
Rule: executeUpdate
Category: ALL_CATEGORIES
Description: Using executeUpdate without parameter binding
Source File: C:\wsast\examples\JavaVulnerableLab-master\src\main\java\org\cysecurity\cspf\jvl\controller\Register.java (line 58)
stmt.executeUpdate("INSERT into users(username, password, email, About,avatar,privilege,secretquestion,secret) values ('"+user+"','"+pass+"','"+email+"','"+about+"','default.jpg','user',1,'"+secret+"')");
...
Explorer the Dataflow Analyzer which forms the core capability of wSAST.
Learn moreLearn more about the Static Analysis capabilities.
Learn moreDiscover the powerful code graphing capabilities which can aid you in code exploration.
Learn moreSee how code searching can help you quickly determine possible paths to exploitation.
Learn more